Integrates Seamlessly with UI
API is designed to make the process of integrating data sources to UI components seamless, tying API request state to UI states automatically.
For example adding an API behavior to an input
will occur oninput
, while a button
, will query the server onclick
.
Preserve Templated URLs
API helps you decouple URLs from your code. Use named API actions like get followers
instead of URLs like http://foo.com/get/1.0/followers.json
in your code.
Centrally manage your entire API making sure you aren't caught modifying urls across your codebase. Define your endpoints using an intuitive templating system that automatically passes data found in your UI.
HTTP 200 is Not Success
Parse your JSON for success
conditions before callbacks fire, making sure server errors caught correctly, still trigger error conditions in your front end code.
Translate APIs on the Fly
Using a third party API that uses some unruly code? Not a problem! API lets you modify an APIs raw JSON response before it is consumed by your code.
Tools for Third-Party Integrations & Mocking
New powerful callbacks like response
and responseAsync
let you asynchronously mock responses and trigger the same callbacks as your API.
API Example
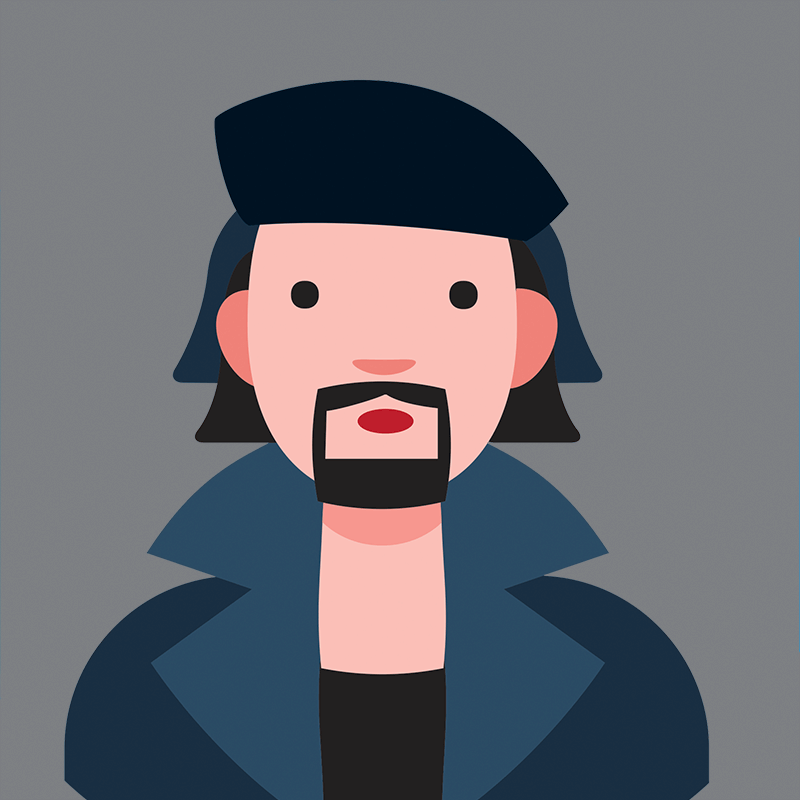
Creating an API
API Actions
API works by defining a set of server actions which interface elements can query. Actions are usually represented by short phrases, things like save profile
, or get followers
which correspond to a templated URL resource on the server.
URL variables specified in actions are substituted at run-time allowing individual components to query different URLs.
URLs listed in your API can include required parameters and optional parameters which may be adjusted for each call.
Required Parameters
{variable}
Optional Parameters
{/variable}
Creating your API
You should define your endpoints once in your application. Usually this is done in a central configuration file included on each page.
These named API endpoints are stored globally in $.fn.api.settings.api
.
Keeping your endpoints defined in one place makes sure when you update your application you will only need to update a single location with new URLs.
Using URLs
Named API actions are not required to use API, you can also manually specify the URL for a request and use the same templating:
Querying API Actions
Attaching API Events
API events are triggered by attaching named actions to elements on your page. These actions look up named endpoints in your API translating templated values from your element for each call.
Any element can have an API action attached directly to it. By default the action will occur on the most appropriate event for the type of element. For example a button will call your server onclick
, an input oninput
, or a form onsubmit
.
API actions and data can be specified in Javascript on initialization:
API actions and data can also be specified in metadata:
Specifying DOM Events
If you need to override what action an API event occurs on you can use the on
parameter.
Calling Immediately
If you require API action to occur immediately use on: 'now'
. This will still trigger the same state updates to the invoked element, but will occur immediately.
Keep in mind passing a new settings object will destroy the previous instance, and all its settings and events. If you want to preserve the previous instance, you can trigger a new request with the query
behavior.
Setting-up Requests
Routing Data to URLs
If your API URLs include templated variables they will be replaced during your request by one of four possible ways, listed in of inheritance.
All parameters used in a URL are encoded using encodeURIComponent
by default, to prevent from malicious strings from affecting your query. To disable this feature you can set encodeParameters: false
.
1. Automatically Routed URL Variables
Some special values will be automatically replaced if specified in URL actions.
Variable | Description | Available for |
---|---|---|
text | current text value of element | All elements |
value | current input value of element | Input elements |
2. URL Variables Specified in Data Attributes
You can include URL values as HTML5 metadata attributes.
This is often the easiest way to include unique URL data for each triggering element. For example, many follow buttons will trigger the same endpoint, but each will have its own user id.
3. Settings Specified in Javascript
URL variables and GET/POST data can be specified at run-time in the Javascript object.
4. Settings Returned from beforeSend
All run settings, not just URL data, can be adjusted in a special callback beforeSend
which occurs before the API request is sent.
Adjusting Requests
Modifying XHR
An additional callback beforeXHR
lets you modify the XHR object before sending. This is useful for adjusting properties of the XHR request like modifying headers, before sending a request.
Disabling Requests
As a convenience, API will automatically prevent requests from occurring on elements that are currently disabled.
Cancelling Requests
BeforeSend can also be used to check for special conditions for a request to be made. If the beforeSend
callback returns false, the request will be cancelled.
Passing Data
1. Routed Form Data
When you use the serializeForm
setting or attach API events on a form, API will automatically include the closest form in data sent to the server.
Structured form data is beneficial over jQuery's serialize for several reasons:
- Serialize Object correctly converts structured form names like
name="name[first]"
into nested object literals. - Structured form data can be modified in Javascript in
beforeSend
. - Form data will automatically be converted to their Javascript equivalents, for instance, checkboxes will be converted to
boolean
values.
Structured Data Example
The following form shows some of the advantages of structured form data mentioned above.
2. Data Routed in Javascript
Server data can be specified directly when initializing an API requests.
3. Data Added in beforeSend
POST or GET data can be specified using a special callback beforeSend
, which can be used to retrieve data before sending a request.
Server Responses
Response Callbacks
Successful responses from the server will trigger onSuccess
, invalid results onFailure
.
onError
will only trigger on XHR errors, but not on invalid JSON responses.
You can use the onResponse
callback to adjust the JSON response before being parsed against a success test.
Determining JSON Success
API has special success conditions for JSON responses. Instead of providing success and failure callbacks based on the HTTP response of the request, a request is considered successful only if the server's response tells you the action was successful. The response is passed to a validation test successTest
which can be used to check the JSON for a valid response.
For example, you might expect all successful JSON responses to return a top level property signifying the success of the response:
You can specify a success test to check for this success
value. This most likely will be set globally for all API requests.
Modifying Response JSON
Since version 2.0, API includes an onResponse
callback which lets you adjust a server's response before a response is validated, allowing you to transform your response before other callbacks fire. This is useful for situations where an API response cannot be modified, but you need the response to conform with a required JSON structure.
Controlling State
UI State
API will automatically add class names for loading
and error
. This will let you trigger different UI states automatically as an API call progresses.
If you need a different element than the triggering API element to receive state class names, you can specify a different selector using settings.stateContext
.
Using stateContext
allows you to easily do things like trigger a loading state on a form when a submit button is pressed.
States Included in API Module
State | Description | API event |
---|---|---|
loading | Indicates a user needs to wait | XHR has initialized |
error | Indicates an error has occurred | XHR Request returns error (does not trigger onAbort caused by page change, or if successTest fails). Stays visible for settings.errorDuration |
disabled | prevents API action | none |
Text State
Initializing an API action with the state module gives you more granular control over UI states, like setting an activated or de-activated state and the ability to adjust text values for each state:
States Included in State Module
State | Description | Occurs on |
---|---|---|
inactive | Default state | |
active | Selected state | Toggled on succesful API request |
activate | Explains activating action | On hover if inactive |
deactivate | Explains deactivating action | On hover if active |
hover | Explains interaction | On hover in all states, overrides activate/deactivate |
disabled | Indicates element cannot be interacted | Triggered programatically. Blocks API requests. |
flash | Text-only state used to display a temporary message | Triggered programatically |
success | Indicates user action was a success | Triggered programatically |
warning | Indicates there was an issue with a user action | Triggered programatically |
Advanced Use
Fulfilling Responses
Since version 2.0, API includes two new parameter response
and responseAsync
which allows you to specify a Javascript object or a function for returning an API response. (These were previously mockResponse
and mockResponseAsync
.)
Using Custom Backends
Using responseAsync
you can specify a function which can execute your API request. This allows for you to use custom backends or wrappers outside of $.ajax
for integrating API requests.
Behavior
All the following behaviors can be called using the syntax:
Behavior | Description |
---|---|
query | Execute query using existing API settings |
add url data(url, data) | Adds data to existing templated url and returns full url string |
get request | Gets promise for current API request |
abort | Aborts current API request |
reset | Removes loading and error state from element |
was cancelled | Returns whether last request was cancelled |
was failure | Returns whether last request was failure |
was successful | Returns whether last request was successful |
was complete | Returns whether last request was completed |
is disabled | Returns whether element is disabled |
is mocked | Returns whether element response is mocked |
is loading | Returns whether element is loading |
set loading | Sets loading state to element |
set error | Sets error state to element |
remove loading | Removes loading state to element |
remove error | Removes error state to element |
get event | Gets event that API request will occur on |
get url encoded value(value) | Returns encodeURIComponent value only if value passsed is not already encoded
|
read cached response(url) | Reads a locally cached response for a URL |
write cached response(url, response) | Writes a cached response for a URL |
create cache | Creates new cache, removing all locally cached URLs |
destroy | Removes API settings from the page and all events |
API
AJAX
API
Default | Description | |
---|---|---|
on | auto | When API event should occur |
cache | true | Can be set to 'local' to cache successful returned AJAX responses when using a JSON API. This helps avoid server roundtrips when API endpoints will return the same results when accessed repeatedly. Setting to false , will add cache busting parameters to the URL. |
stateContext | this | UI state will be applied to this element, defaults to triggering element. |
encodeParameters | true | Whether to encode parameters with encodeURIComponent before adding into url string |
defaultData | true | Whether to automatically include default data like {value} and {text} |
serializeForm | false | Whether to serialize closest form and include in request |
throttle | 0 | How long to wait when a request is made before triggering request, useful for rate limiting oninput |
throttleFirstRequest | true | When set to false will not delay the first request made, when no others are queued |
interruptRequests | false | Whether an API request can occur while another request is still pending |
loadingDuration | 0 | Minimum duration to show loading indication |
hideError | auto | The default auto will automatically remove error state after error duration, unless the element is a form |
errorDuration | 2000 | Setting to true , will not remove error. Setting to a duration in milliseconds to show error state after request error. |
Request Settings
Default | Description | Possible Values | |
---|---|---|---|
action | false | Named API action for query, originally specified in $.fn.settings.api | String or false |
url | false | Templated URL for query, will override specified action | String or false |
urlData | false | Variables to use for replacement | |
response | false | Can be set to a Javascript object which will be returned automatically instead of requesting JSON from server | {} or false |
responseAsync(settings, callback) | false | When specified, this function can be used to retrieve content from a server and return it asynchronously instead of a standard AJAX call. The callback function should return the server response. | function or false |
mockResponse | false | Alias of response |
|
mockResponseAsync | false | Alias of responseAsync |
|
method | get | Method for transmitting request to server | Any valid http method |
dataType | JSON | Expected data type of response | xml, json, jsonp, script, html, text |
data | {} | POST/GET Data to Send with Request |
Callbacks
Context | Description | |
---|---|---|
beforeSend(settings) | initialized element | Allows modifying settings before request, or cancelling request |
beforeXHR(xhrObject) | Allows modifying XHR object for request | |
onRequest(promise, xhr) | state context | Callback that occurs when request is made. Receives both the API success promise and the XHR request promise. |
onResponse(response) | state context | Allows modifying the server's response before parsed by other callbacks to determine API event success |
successTest(response) | Determines whether completed JSON response should be treated as successful | |
onSuccess(response, element, xhr) | state context | Callback after successful response, JSON response must pass successTest |
onComplete(response, element, xhr) | state context | Callback on request complete regardless of conditions |
onFailure(response, element) | state context | Callback on failed response, or JSON response that fails successTest |
onError(errorMessage, element, xhr) | state context | Callback on server error from returned status code, or XHR failure. |
onAbort(errorMessage, element, xhr) | state context | Callback on abort caused by user clicking a link or manually cancelling request. |
Module
These settings are native to all modules, and define how the component ties content to DOM attributes, and debugging settings for the module.
Default | Description | |
---|---|---|
name | API | Name used in log statements |
namespace | api | Event namespace. Makes sure module teardown does not effect other events attached to an element. |
regExp |
regExp : {
required: /\{\$*[A-z0-9]+\}/g,
optional: /\{\/\$*[A-z0-9]+\}/g,
}
|
Regular expressions used for template matching |
selector |
selector: {
disabled : '.disabled',
form : 'form'
}
|
Selectors used to find parts of a module |
className |
className: {
loading : 'loading',
error : 'error'
}
|
Class names used to determine element state |
metadata |
metadata: {
action : 'action',
url : 'url'
}
|
Metadata used to store XHR and response promise |
silent | False | Silences all console output including error messages, regardless of other debug settings. |
debug | false | Debug output to console |
performance | true | Show console.table output with performance metrics |
verbose | false | Debug output includes all internal behaviors |
errors |
// errors
error : {
beforeSend : 'The before send function has aborted the request',
error : 'There was an error with your request',
exitConditions : 'API Request Aborted. Exit conditions met',
JSONParse : 'JSON could not be parsed during error handling',
legacyParameters : 'You are using legacy API success callback names',
missingAction : 'API action used but no url was defined',
missingSerialize : 'Required dependency jquery-serialize-object missing, using basic serialize',
missingURL : 'No URL specified for API event',
noReturnedValue : 'The beforeSend callback must return a settings object, beforeSend ignored.',
parseError : 'There was an error parsing your request',
requiredParameter : 'Missing a required URL parameter: ',
statusMessage : 'Server gave an error: ',
timeout : 'Your request timed out'
}
|