Introduction
Visibility provides a set of callbacks that can be attached to any element and trigger when a specific visibility event occurs.
There are a variety of uses for attaching events to visibility. Here are some of the more common ones.
Usage
How To Use
Visibility provides a set of callbacks which can be used to attach events to an element's position on screen.
Each scroll change will trigger an animation frame request that will fire callbacks for an element.
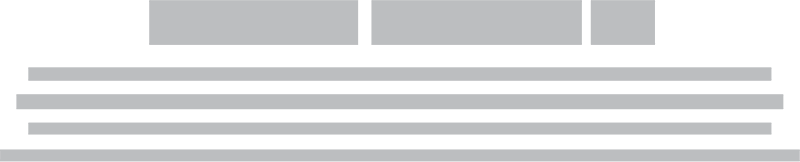

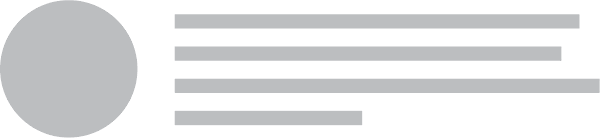
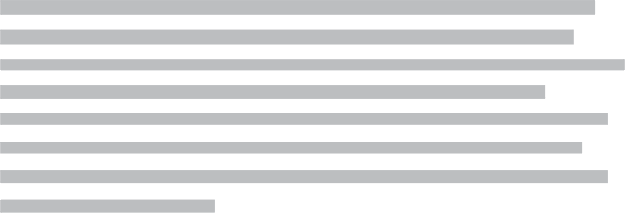
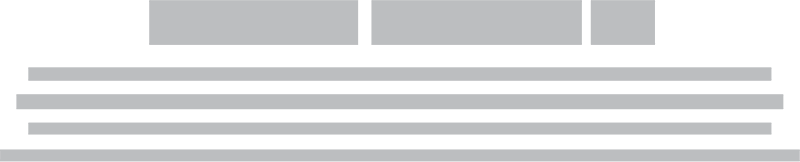

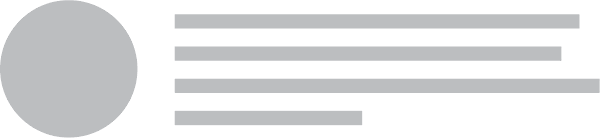
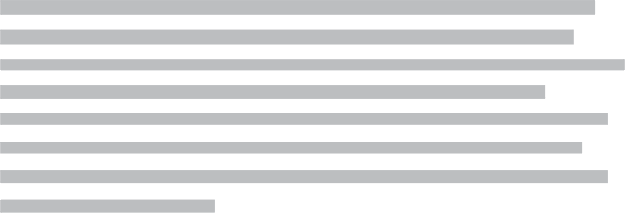

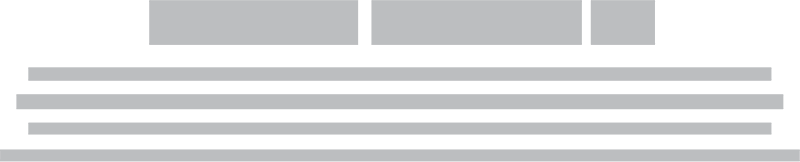

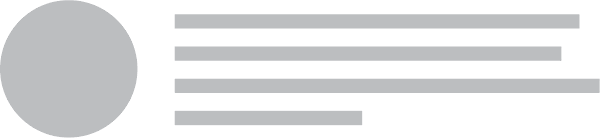
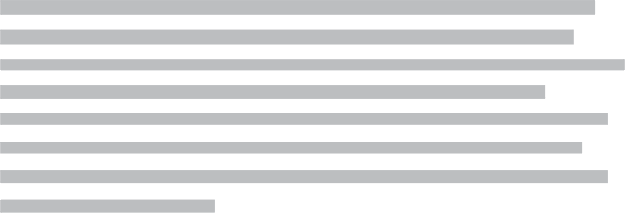

Calculation | Value |
---|---|
pixelsPassed | |
percentagePassed | |
fits | |
width | |
height | |
onScreen | |
offScreen | |
passing | |
topVisible | |
bottomVisible | |
topPassed | |
bottomPassed |
Changing Callback Frequency
Visibility's default settings will only have each callback occur the first time which the conditions are met. On subsequent occurences the event will not fire.
Setting continuous: true
will make the callback fire anytime the callback conditions are met. So for example if you set a "top visible" callback, this callback will fire with each change in scroll when the top is visible on the page.
Setting once: false
will make the callback fire each time a callback becomes true. So, using the same "top visible" example, the callback will fire each time the top of an element is passed. Even if you scroll back up and pass the element again
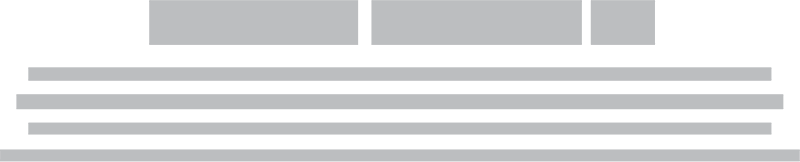

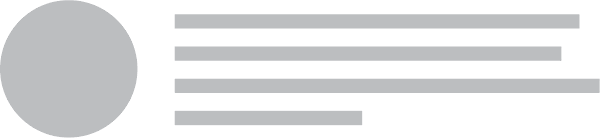
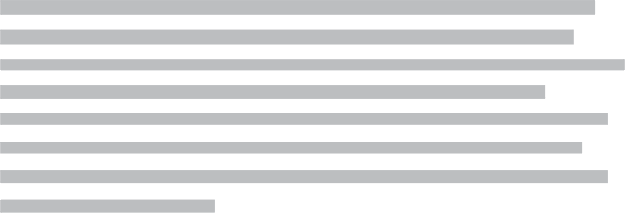
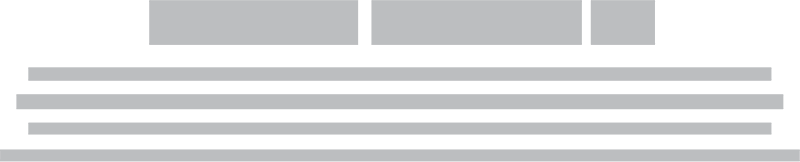

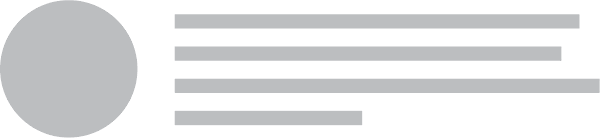
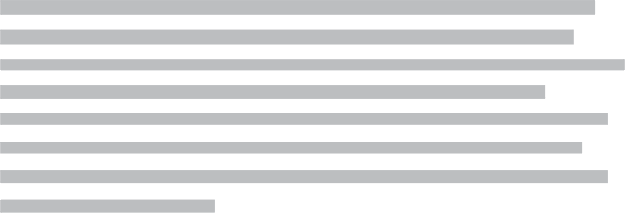

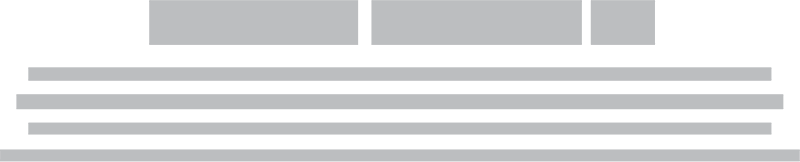

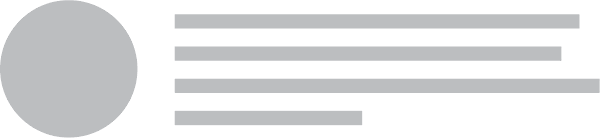
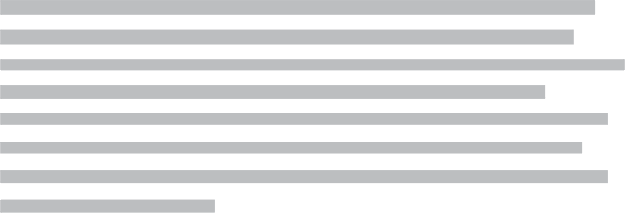

Callbacks
Callbacks are separated into two categories. Standard events will occur the first animation frame where the conditions evaluated to true.
Standard Events
Event | Occurs | Pseudocode |
---|---|---|
onOnScreen | Any part of element is in current scroll viewport |
screen.bottom >= element.top && screen.bottom <= element.bottom
|
onOffScreen | No part of element is in current scroll viewport |
screen.bottom <= element.top || screen.top >= element.bottom
|
onTopVisible | Element's top edge has passed bottom of screen |
screen.bottom >= element.top
|
onTopPassed | Element's top edge has passed top of the screen |
screen.top >= element.top
|
onBottomVisible | Element's bottom edge has passed bottom of screen |
screen.bottom >= element.top
|
onPassing | Any part of an element is visible on screen |
screen.bottom >= element.top && screen.top < element.bottom
|
onBottomPassed | Element's bottom edge has passed top of screen |
screen.top >= element.bottom
|
Grouped Events
onPassed
allows you to specify a collection of callbacks that occur after different percentages or pixels of an element are passed
Event | Occurs | Example |
---|---|---|
onPassed {} | A distance from the top of an element's content has been passed, either as a percentage or in pixels |
onPassed: {
40: function() {
// do something when having passed 40 pixels.
},
'80%': function() {
// do something at 80%
}
}
|
Reverse Events
Reverse events will occur under the same conditions but as a user scrolls back up the page.
Event | Occurs | Pseudocode |
---|---|---|
onTopVisibleReverse | Element's top edge has not passed bottom of screen |
screen.bottom >= element.top
|
onTopPassedReverse | Element's top edge has not passed top of the screen |
screen.top >= element.top
|
onBottomVisibleReverse | Element's bottom edge has not passed bottom of screen |
screen.bottom >= element.top
|
onPassingReverse | Element's top has not passed top of screen but bottom has |
screen.bottom >= element.top && screen.top < element.bottom
|
onBottomPassedReverse | Element's bottom edge has not passed top of screen |
screen.top >= element.bottom
|
Behaviors
Visibility includes several useful behaviors for interacting with the component
Behavior | Usage |
---|---|
disable callbacks | Disable callbacks temporarily. This is useful if you need to adjust scroll position and do not want to trigger callbacks during the position change. |
enable callbacks | Re-enable callbacks |
is on screen | Returns whether element is on screen |
is off screen | Returns whether element is off screen |
get pixels passed | Returns number of pixels passed in current element from top of element |
get element calculations | Returns element calculations as object |
get screen calculations | Returns screen calculations as object |
get screen size | Returns screen size as object |
Examples
Infinite Scroll
As an alternative to pagination you can use onBottomVisible
to load content automatically when the bottom of a container is reached.
Infinite Scroll Example
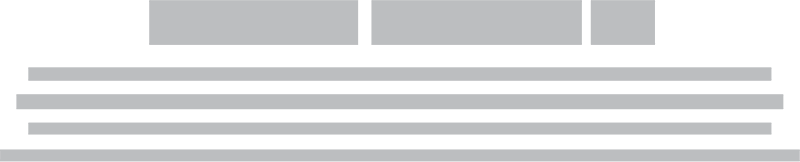

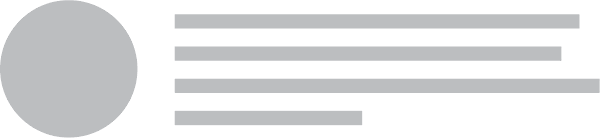

Lazy Loading Images
Visibility includes several shortcuts for setting up common visibility events. Using the setting type: 'image'
will automatically attach events to an images topVisible
to load the contents of data-src
as src
.
You can specify a placeholder image as the current src
to make sure content doesn't jump when an image loads, or you can specify no placeholder and have the image appear.
By default images will appear without animation, however you can also specify a named transition, and a duration that should be used for animating the image into view
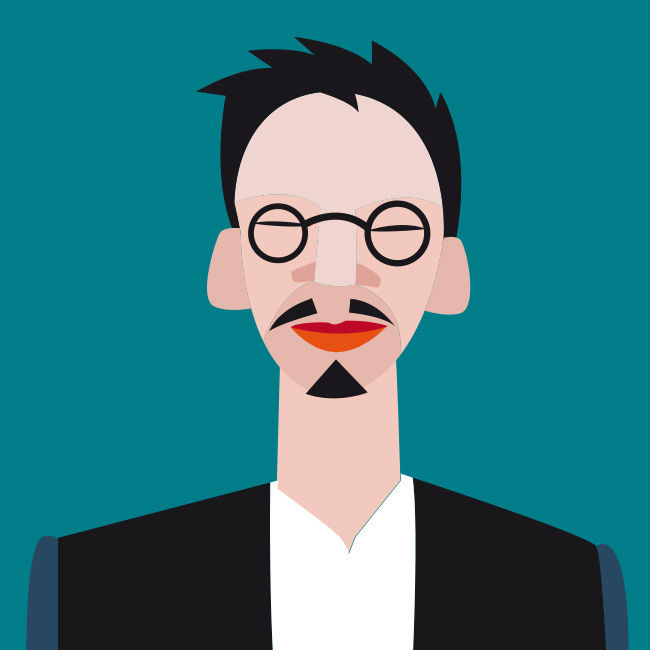
Elliot Fu

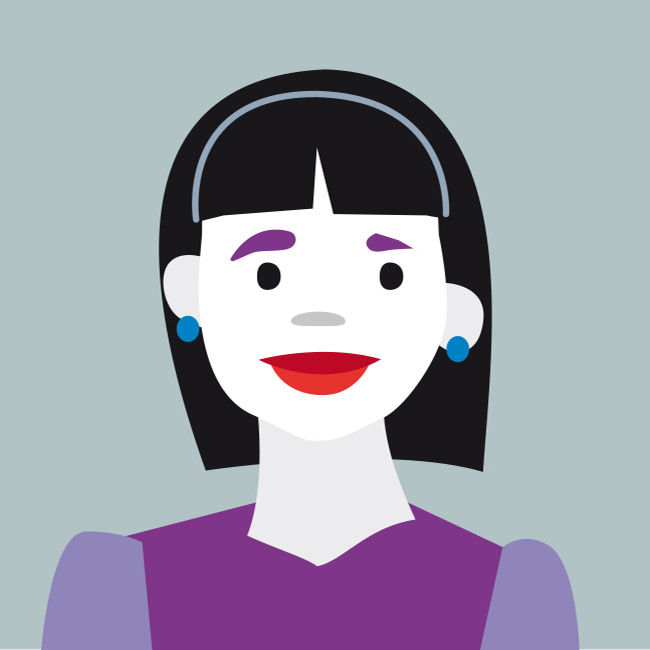
Helen Troy

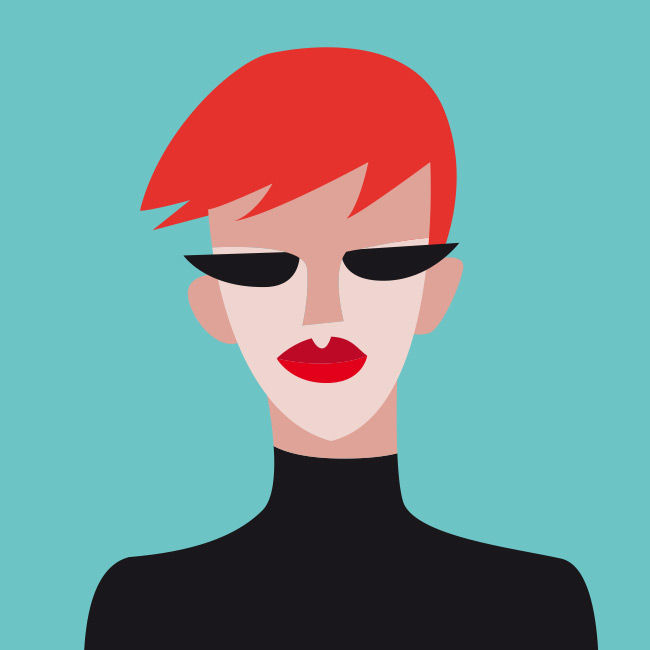
Jenny Hess

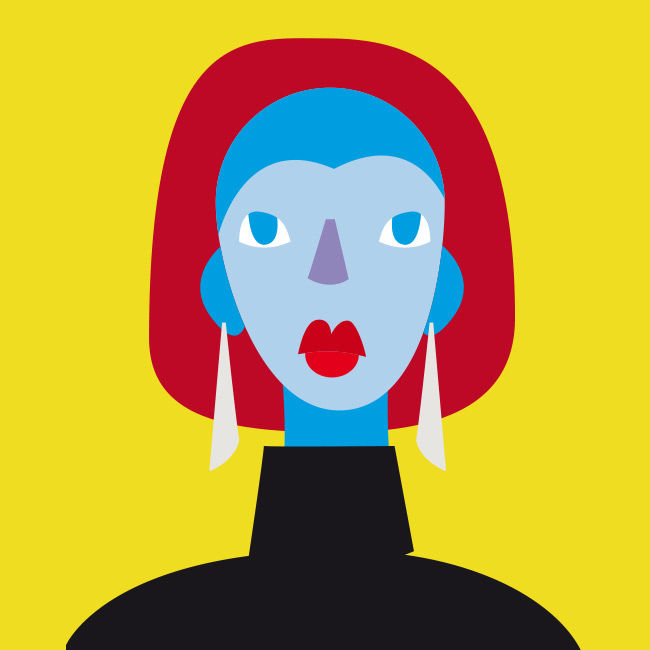
Veronika Ossi

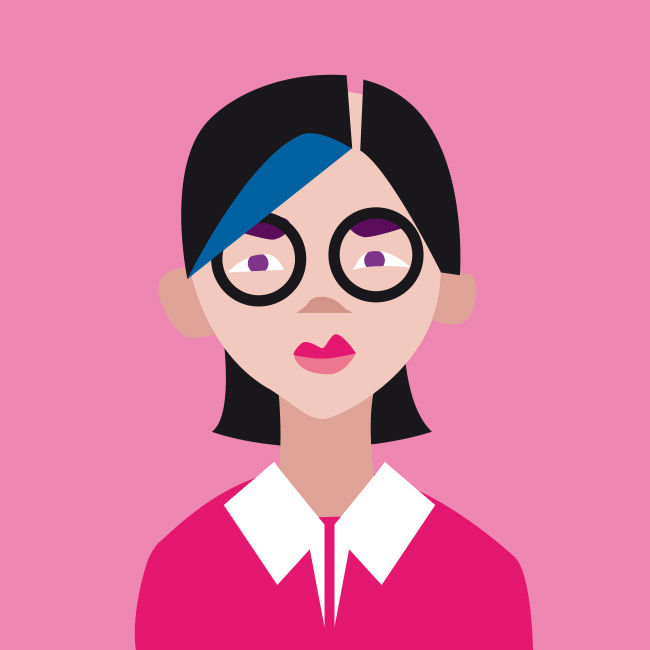
Stevie

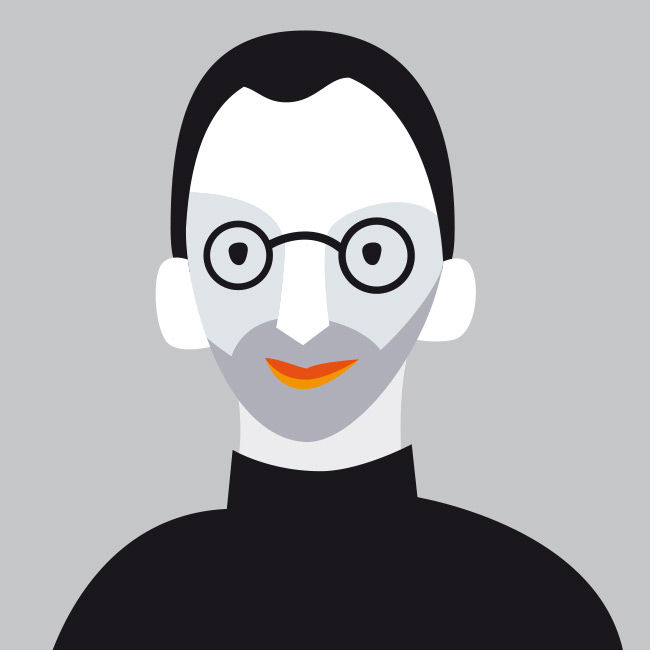
Steve Jobes

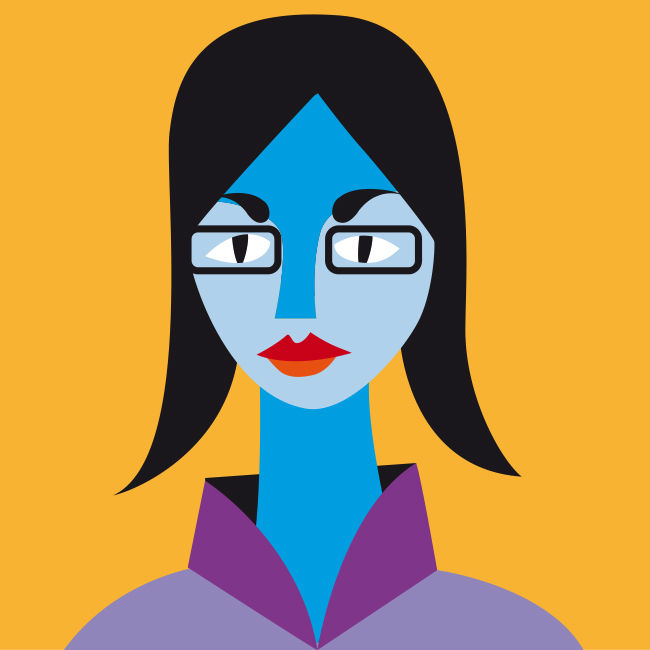
Ade

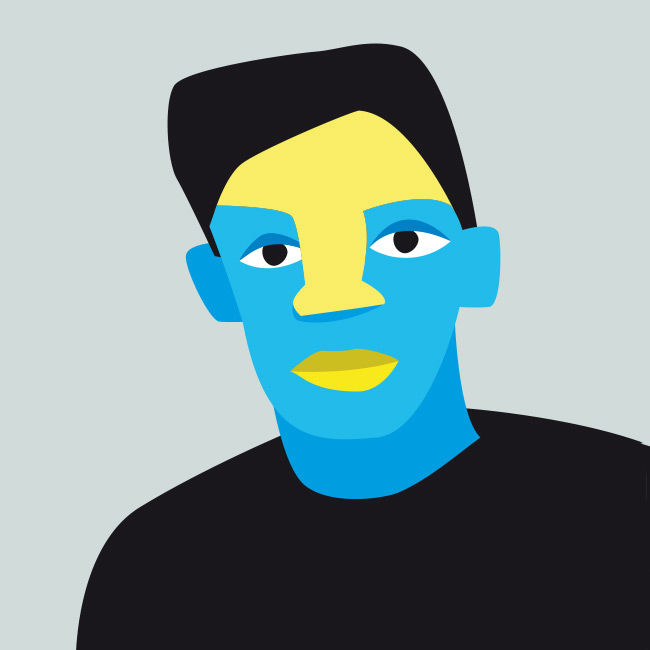
Chris

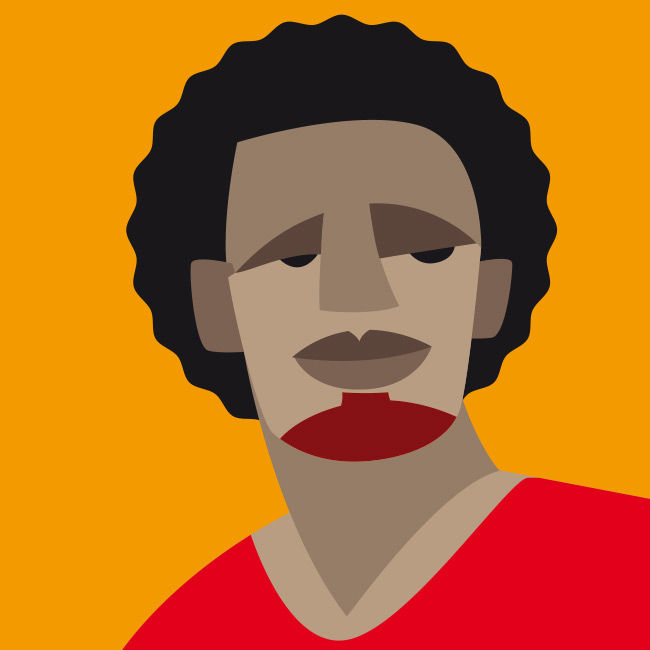
Joe Henderson

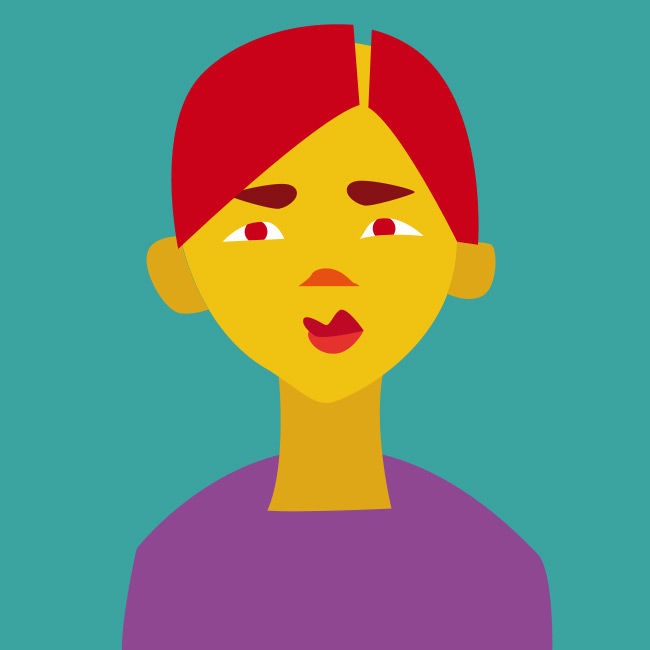
Laura

Gradual Changes
Each callback receives all calculated values as its first parameter, allowing you to adjust an element using
Settings
Functionality
Settings to configure visibility behavior
Setting | Default | Description |
---|---|---|
once | true | When set to false a callback will occur each time an element passes the threshold for a condition. |
continuous | false | When set to true a callback will occur anytime an element passes a condition not just immediately after the threshold is met. |
type | false | Set to image to load images when on screen. Set to fixed to add class name fixed when passed. |
initialCheck | true | Whether visibility conditions should be checked immediately on init |
context | window | The scroll context visibility should use. |
refreshOnLoad | true | Whether visibility conditions should be checked on window load . This ensures that after images load content positions will be updated correctly. |
refreshOnResize | true | Whether visibility conditions should be checked on window resize . Useful when content resizes causes continuous changes in position |
checkOnRefresh | true | Whether visibility conditions should be checked on calls to refresh . These calls can be triggered from either resize , load or manually calling $('.foo').visibility('refresh') |
zIndex | 1 | Specify a z-index when using type: 'fixed' . New in 2.2 |
offset | 0 | Value that context scrollTop should be adjusted in pixels. Useful for making content appear below content fixed to the page. |
includeMargin | false | Whether element calculations should include its margin |
throttle | false | When set to an integer, scroll position will be debounced using this ms value. false will debounce with requestAnimationFrame . |
observeChanges | true | Whether to automatically refresh content when changes are made to the element's DOM subtree |
transition | false | When using type: image allows you to specify transition when showing a loaded image |
duration | 1000 | When using type: image allows you to specify transition duration |
Visibility Callbacks
Callbacks that occur on named visibility events
Context | Description | |
---|---|---|
onTopVisible | $element | Element's top edge has passed bottom of screen |
onTopPassed | $element | Element's top edge has passed top of the screen |
onBottomVisible | $element | Element's bottom edge has passed bottom of screen |
onPassing | $element | Any part of an element is visible on screen |
onBottomPassed | $element | Element's bottom edge has passed top of screen |
onTopVisibleReverse | $element | Element's top edge has not passed bottom of screen |
onTopPassedReverse | $element | Element's top edge has not passed top of the screen |
onBottomVisibleReverse | $element | Element's bottom edge has not passed bottom of screen |
onPassingReverse | $element | Element's top has not passed top of screen but bottom has |
onBottomPassedReverse | $element | Element's bottom edge has not passed top of screen |
Image Callbacks
New in 2.2
Callbacks that occur only when using type: 'fixed'
Context | Description | |
---|---|---|
onLoad | img | Occurs after an image has completed loading |
onAllLoaded | last loaded img | Occurs after all img initialized at the same time have loaded. |
Fixed Callbacks
New in 2.2
Callbacks that occur only when using type: 'fixed'
Context | Description | |
---|---|---|
onFixed | $element | Occurs after element has been assigned position fixed |
onUnfixed | $element | Occurs after element has been removed from fixed position |
Utility Callbacks
Callbacks that occur on named visibility events
Context | Description | |
---|---|---|
onUpdate(calculations) | $element | Occurs each time an elements calculations are updated |
onRefresh | $element | Occurs whenever element's visibility is refreshed |
DOM Settings
DOM settings specify how this module should interface with the DOM
Setting | Default | Description |
---|---|---|
namespace | visibility | Event namespace. Makes sure module teardown does not effect other events attached to an element. |
className |
className : {
fixed : 'fixed',
}
|
Class names used to attach style to state |
Debug Settings
Debug settings controls debug output to the console
Setting | Default | Description |
---|---|---|
name | Visibility | Name used in debug logs |
silent | False | Silences all console output including error messages, regardless of other debug settings. |
debug | False | Provides standard debug output to console |
performance | True | Provides standard debug output to console |
verbose | True | Provides ancillary debug output to console |
errors |
error : {
method : 'The method you called is not defined.',
}
|