We have more to share with you. Follow us along to modal 2
That's everything!
Your inbox is getting full, would you like us to enable automatic archiving of old messages?
Are you sure you want to delete your account
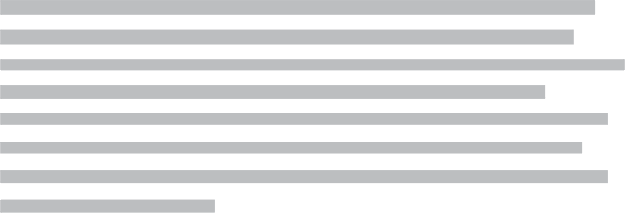
Are you sure you want to delete your account
Are you sure you want to delete your account
Give us your feedback
Do you want to change that thing to something else?
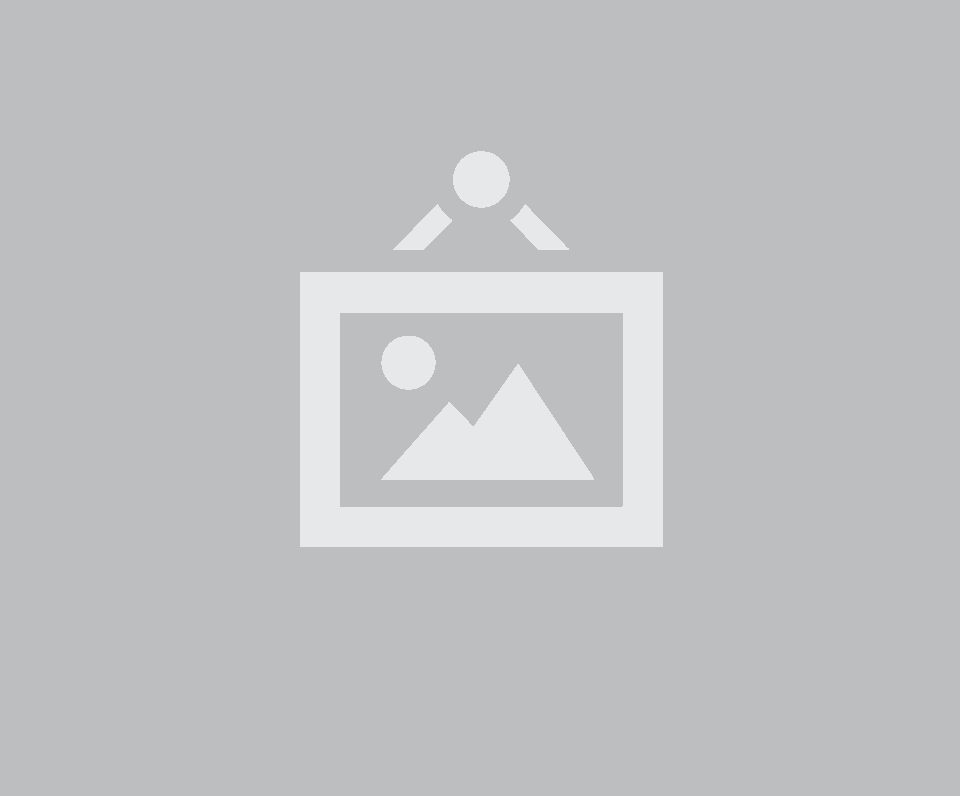
This is an example of expanded content that will cause the modal's dimmer to scroll
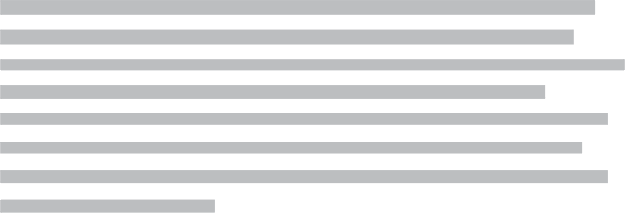
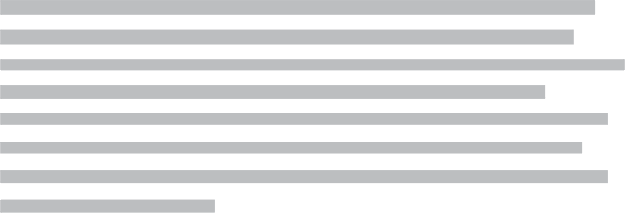
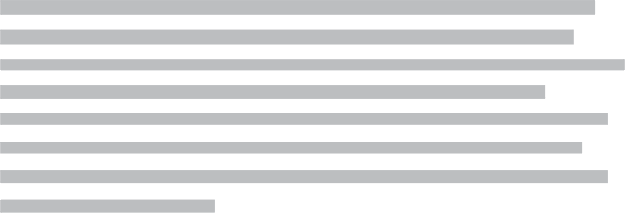
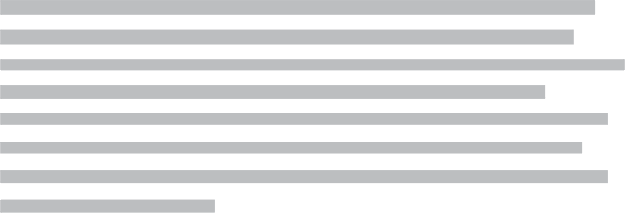
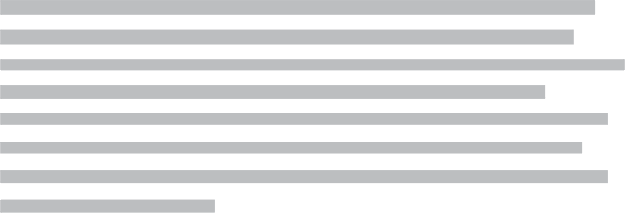
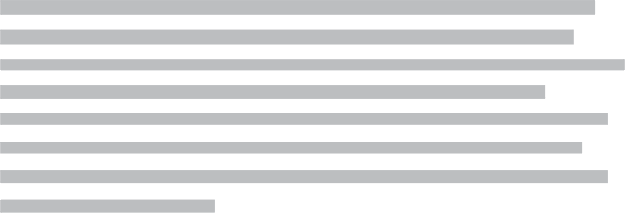
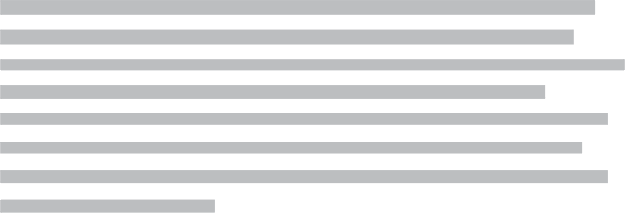
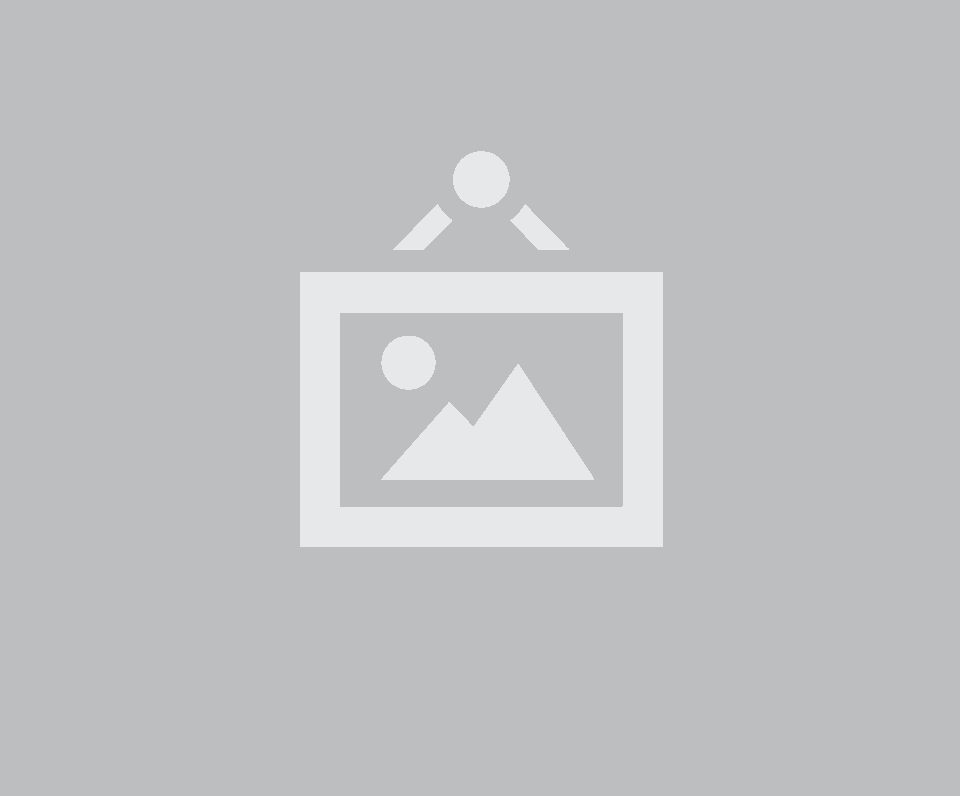
This is an example of expanded content that will cause the modal's dimmer to scroll
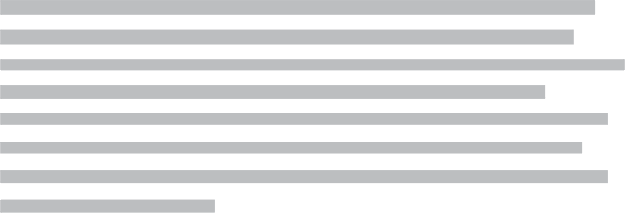
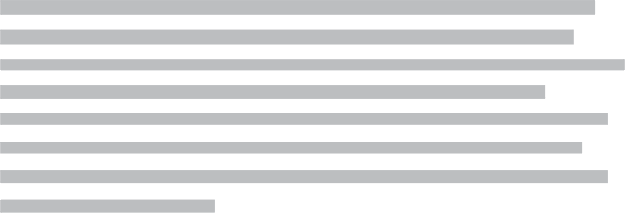
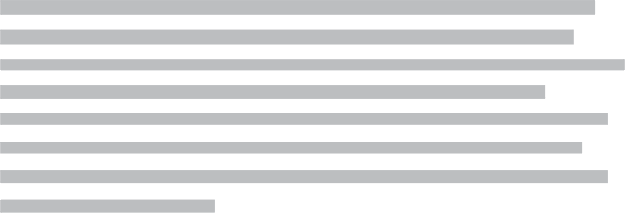
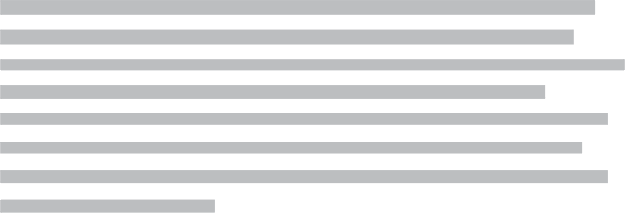
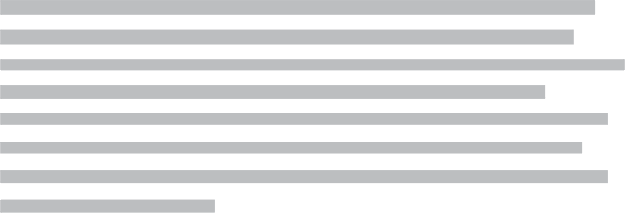
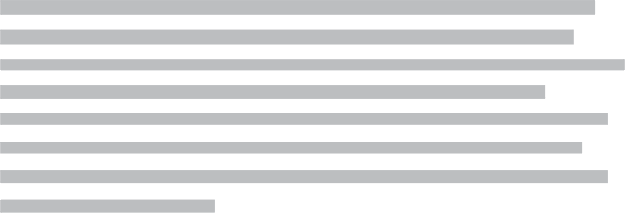
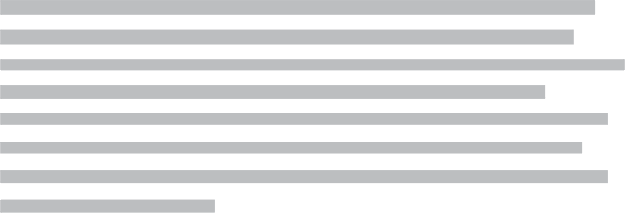
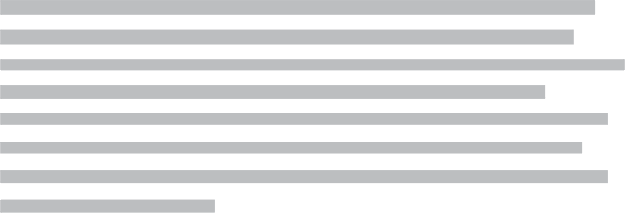
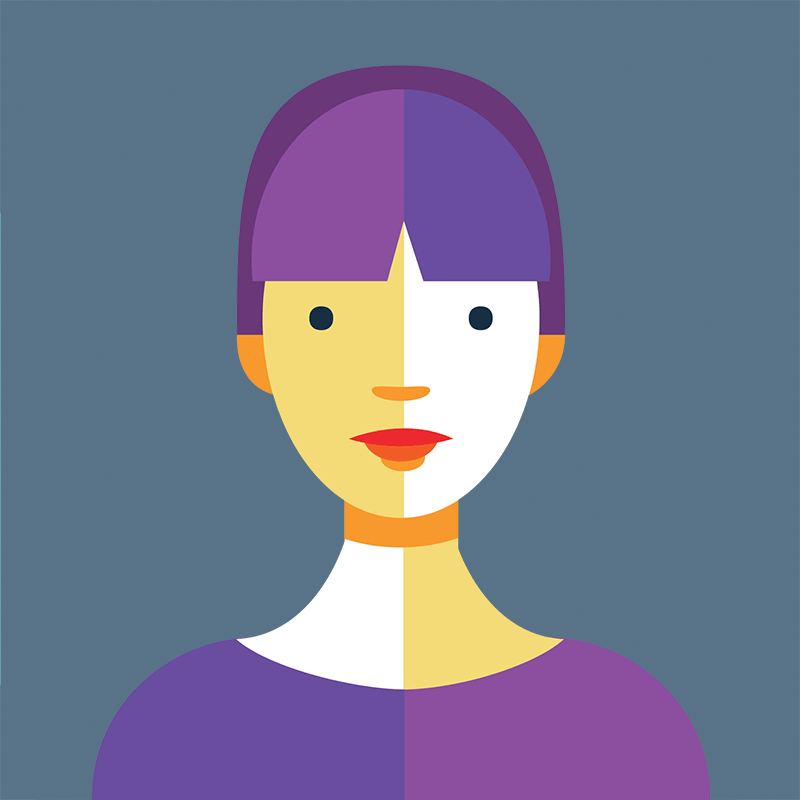
We've found the following gravatar image associated with your e-mail address.
Is it okay to use this photo?
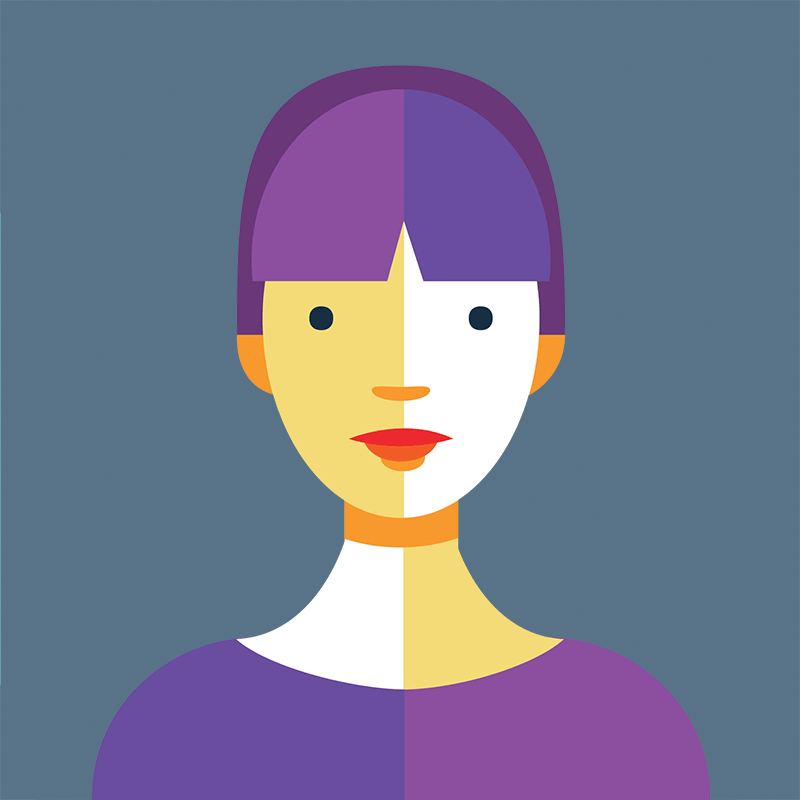
We've found the following gravatar image associated with your e-mail address.
Is it okay to use this photo?
Types
Modal
A standard modal
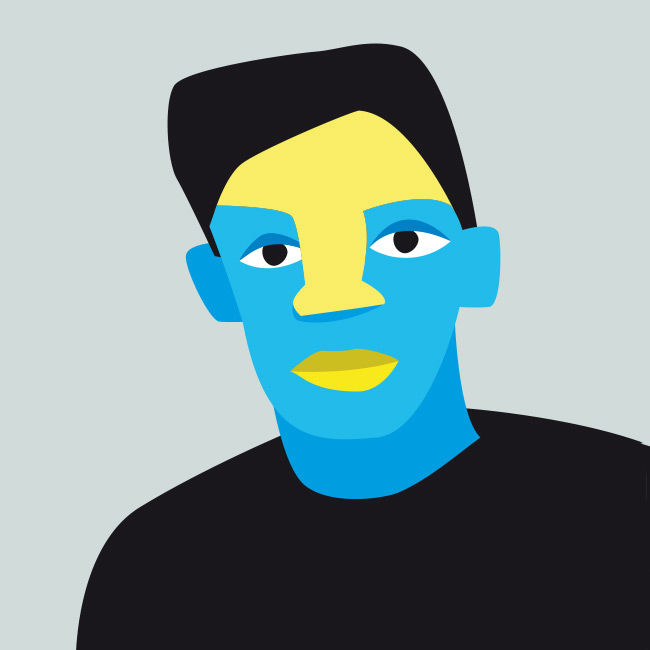
We've grabbed the following image from the gravatar image associated with your registered e-mail address.
Is it okay to use this photo?
Basic
A modal can reduce its complexity
Your inbox is getting full, would you like us to enable automatic archiving of old messages?
Content
Header
A modal can have a header
Content
A modal can contain content
Image Content New in 2.0
A modal can contain image content
Actions
A modal can contain a row of actions
Variations
Full Screen
A modal can use the entire size of the screen
Size
A modal can vary in size
Scrolling Content
New in 2.2.11
A modal can use the entire size of the screen
Very long content goes here
States
Active
An active modal is visible on the page
Examples
Disabling Vertical Centering
New in 2.3
When your modal has dynamic content, or multiple steps, it can make sense to disable centering so content doesn't jump around vertically when its height changes.
Scrolling Modal
When your modal content exceeds the height of the browser the scrollable area will automatically expand to include just enough space for scrolling, without scrolling the page below.
Internally Scrolling Content
You may prefer to have the content of your modal scroll itself, you can do this by using the scrolling content
variation.
Multiple Modals
A modal can open a second modal. If you use allowMultiple: true
parameter the second modal will be opened on top of the first modal. Otherwise the modal will be closed before the second modal is opened.
Forcing a Choice
You can disable a modal's dimmer from being closed by click to force a user to make a choice
Approve / Deny Callbacks
Modals will automatically tie approve deny callbacks to any positive/approve, negative/deny or ok/cancel buttons.
Attach events
A modal can attach events to another element
Transitions
A modal can use any named ui transition.
Dimmer Variations
Modals can specify additional variations like blurring
or inverted
which adjust how the dimmer displays.
Usage
Initializing a modal
A modal can be included anywhere on the page. On initialization a modal's current size will be cached, and the element will be detached from the DOM and moved inside a dimmer.
Behavior
All the following behaviors can be called using the syntax:
Behavior | Description |
---|---|
show | Shows the modal |
hide | Hides the modal |
toggle | Toggles the modal |
refresh | Refreshes centering of modal on page |
show dimmer | Shows associated page dimmer |
hide dimmer | Hides associated page dimmer |
hide others | Hides all modals not selected modal in a dimmer |
hide all | Hides all visible modals in the same dimmer |
cache sizes | Caches current modal size |
can fit | Returns whether the modal can fit on the page |
is active | Returns whether the modal is active |
set active | Sets modal to active |
Settings
Modal Settings
Modal settings modify the modal's behavior
Setting | Default | Description |
---|---|---|
detachable | true | If set to false will prevent the modal from being moved to inside the dimmer |
useFlex | 'auto' | Auto will automatically use flex in browsers that support absolutely positioned elements inside flex containers. Setting to true/false will force this setting for all browsers. |
autofocus | true | When true, the first form input inside the modal will receive focus when shown. Set this to false to prevent this behavior. |
observeChanges | false | Whether any change in modal DOM should automatically refresh cached positions |
allowMultiple | false | If set to true will not close other visible modals when opening a new one |
keyboardShortcuts | true | Whether to automatically bind keyboard shortcuts |
offset | 0 | A vertical offset to allow for content outside of modal, for example a close button, to be centered. |
context | body | Selector or jquery object specifying the area to dim |
closable | true | Setting to false will not allow you to close the modal by clicking on the dimmer |
dimmerSettings |
{
closable : false,
useCSS : true
}
|
You can specify custom settings to extend UI dimmer |
transition | scale | Named transition to use when animating menu in and out, full list can be found in ui transitions docs. |
duration | 400 | Duration of animation |
queue | false | Whether additional animations should queue |
Callbacks
Callbacks specify a function to occur after a specific behavior.
Setting | Context | Description |
---|---|---|
onShow | Modal | Is called when a modal starts to show. |
onVisible | Modal | Is called after a modal has finished showing animating. |
onHide($element) | Modal | Is called after a modal starts to hide. If the function returns false , the modal will not hide. |
onHidden | Modal | Is called after a modal has finished hiding animation. |
onApprove($element) | Click | Is called after a positive, approve or ok button is pressed. If the function returns false , the modal will not hide. |
onDeny($element) | Modal | Is called after a negative, deny or cancel button is pressed. If the function returns false the modal will not hide. |
DOM Settings
DOM settings specify how this module should interface with the DOM
Setting | Default | Description |
---|---|---|
namespace | modal | Event namespace. Makes sure module teardown does not effect other events attached to an element. |
selector |
selector : {
close : '.close, .actions .button',
approve : '.actions .positive, .actions .approve, .actions .ok',
deny : '.actions .negative, .actions .deny, .actions .cancel'
},
|
|
className |
className : {
active : 'active',
scrolling : 'scrolling'
}
|
Debug Settings
Debug settings controls debug output to the console
Setting | Default | Description |
---|---|---|
name | Modal | Name used in debug logs |
debug | False | Provides standard debug output to console |
performance | True | Provides standard debug output to console |
verbose | True | Provides ancillary debug output to console |
error |
error : {
method : 'The method you called is not defined.'
}
|